In the ever-evolving landscape of technology, developers are constantly striving to create robust and reliable applications. Errors and exceptions are inevitable, and how we handle them can make a significant difference in the user experience and functionality of our software. Python, with its elegant syntax and powerful features, provides a comprehensive mechanism for error handling through the try
and except
statements. In this Technology Moment, we’ll delve into the importance of these constructs, how they work, and why mastering them is crucial for writing resilient Python code. Join us as we explore the art of gracefully managing the unexpected in the world of programming.
Error handling is an essential aspect of programming that ensures your code can gracefully handle unexpected situations. In Python, the try-except block is a fundamental tool for managing errors and exceptions that may arise during the execution of your programs.
The Try Except Python block allows you to write code that attempts to execute a certain operation (within the try block) and gracefully catches and handles any exceptions (within the except block) that might occur. This mechanism not only prevents your program from crashing but also provides a way to give informative feedback to the user or take corrective actions.
Using try-except blocks is like adding a safety net to your code. Just as a safety net catches a trapeze artist who misses a catch, a try-except block catches errors that could disrupt the flow of your program. This makes your code more robust and user-friendly, ensuring that it can handle unexpected events without failing catastrophically.
In this article, we’ll dive deep into the world of try-except blocks in Python. We’ll start with a clear definition and basic syntax, then explore more advanced topics such as handling multiple exceptions, using else and finally clauses, and best practices. By the end, you’ll have a solid understanding of how to use try-except blocks effectively to write more reliable and maintainable Python code.
Whether you’re a seasoned developer or just starting your coding journey, mastering error handling with try-except blocks will elevate your Python programming skills. So, let’s get started and explore the ins and outs of this crucial aspect of Python!
Table of Contents
What is a Try Except Python Block?
A try-except block in Python is a fundamental construct for handling exceptions, which are errors that occur during the execution of a program. The try-except block allows you to test a block of code for errors and provide a response or solution if an error occurs. This way, your program can handle the error gracefully without crashing.
Definition and Basic Syntax
The try-except block consists of two main parts: the try
block and the except
block. Here’s the basic syntax:
try:
# code that might raise an exception
except:
# code that runs if an exception occurs
- try: This keyword is used to define a block of code that you want to monitor for exceptions. You place the code that you suspect might throw an error within this block.
- except: This keyword is used to define a block of code that will execute if an exception occurs in the try block. You can specify different actions depending on the type of exception.
How It Works
When Python encounters a try-except block, it first executes the code inside the try
block. If no errors occur, the except
block is skipped, and the program continues as normal. However, if an error (exception) occurs during the execution of the code in the try
block, Python immediately stops the execution of the try
block and jumps to the except
block. The code inside the except
block is then executed, allowing you to handle the error.
Here’s a simple example :
try:
result = 10 / 0
except ZeroDivisionError:
print("You can't divide by zero!")
In this example:
- The
try
block contains code that attempts to divide 10 by 0, which is not allowed and will raise aZeroDivisionError
. - When the
ZeroDivisionError
occurs, Python jumps to theexcept
block. - The
except
block then prints the message, “You can’t divide by zero!”
This way, the program doesn’t crash when an exception occurs. Instead, it gracefully handles the error by executing the code in the except
block.
Benefits of Using Try-Except Blocks
- Graceful Error Handling: Prevents your program from crashing and allows you to handle errors gracefully.
- Improved User Experience: Users receive informative messages or fallback actions instead of abrupt program termination.
- Debugging Aid: Helps in identifying and debugging the portions of code that may cause errors.
Why Use Try-Except Blocks?
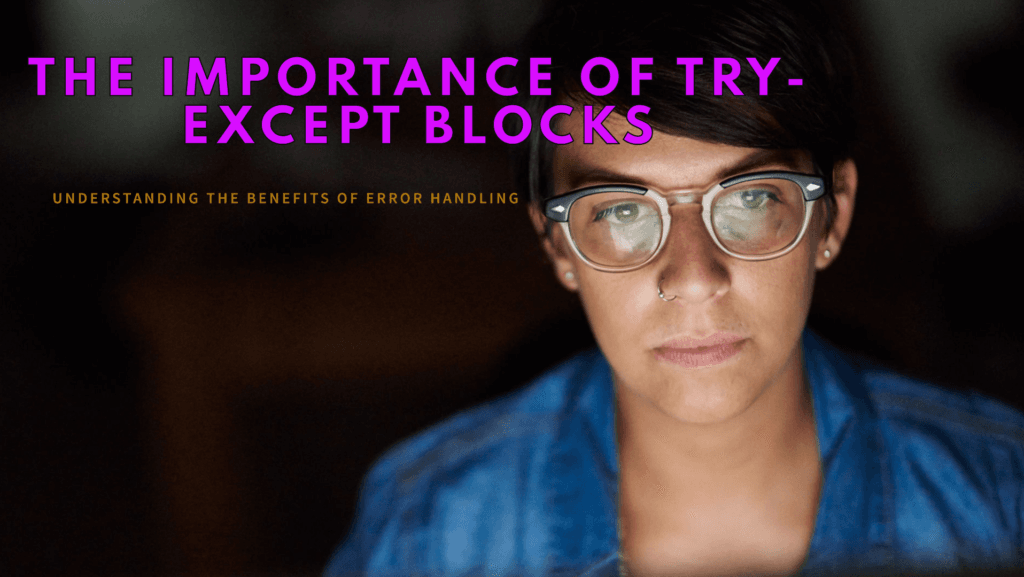
Importance in Robust Programming
Error handling is a critical aspect of programming that ensures your code can deal with unexpected issues gracefully. When an error occurs during the execution of a program, it can cause the program to crash, leading to a poor user experience and potential loss of data. Try-except blocks provide a structured way to handle these errors and keep your program running smoothly.
Here’s why using try-except blocks is so crucial:
- Preventing Program Crashes: One of the primary reasons to use try-except blocks is to prevent your program from crashing. When an error occurs within a try block, the program’s control flow is immediately passed to the corresponding except block, where you can handle the error. This prevents the program from terminating unexpectedly.
- Improving User Experience: By handling errors gracefully, you can provide users with informative messages or alternative solutions rather than showing a generic error message or closing the program. This enhances the overall user experience and makes your software more reliable and user-friendly.
- Maintaining Data Integrity: In many applications, especially those dealing with file operations, database transactions, or network communication, errors can lead to data corruption or loss. Using try-except blocks helps ensure that any issues are managed correctly, allowing you to roll back transactions, close files properly, or retry network requests, thereby maintaining data integrity.
- Debugging and Logging: Try-except blocks allow you to log errors, making it easier to debug your code. By catching exceptions and logging them, you can keep track of what went wrong and where, which is invaluable for debugging and improving your code. This is especially important in production environments where direct access to error output might be limited.
- Flexibility in Error Handling: Python’s try-except mechanism provides flexibility in handling different types of errors. You can catch specific exceptions and handle them accordingly, or you can use a general exception to catch any error. This allows you to tailor your error handling strategy based on the specific needs of your application.
- Code Readability and Maintenance: Using try-except blocks makes your code more readable and easier to maintain. By explicitly defining what should happen when an error occurs, you make the code’s behavior clear to anyone reading or maintaining it.
Examples of Common Errors Caught by Try-Except
To illustrate the importance of try-except blocks, let’s look at some common scenarios where they can be used effectively:
- File Operations: When working with files, numerous things can go wrong, such as the file not existing, lacking the necessary permissions, or encountering an I/O error. By using try-except blocks, you can handle these errors and take appropriate action, such as creating the file, asking for correct permissions, or retrying the operation.
try:
with open('example.txt', 'r') as file:
content = file.read()
except FileNotFoundError:
print("The file does not exist.")
except PermissionError:
print("You do not have permission to read this file.")
2. User Input: When taking user input, especially if it needs to be converted to a specific type (e.g., an integer), you might encounter value errors. Try-except blocks allow you to catch these errors and prompt the user to enter the correct type of data.
try:
user_input = int(input("Enter a number: "))
except ValueError:
print("Please enter a valid number.")
3. Network Requests: When making network requests, you might encounter errors such as timeouts, connection errors, or invalid URLs. Using try-except blocks enables you to handle these situations gracefully, such as retrying the request or providing a fallback option.
import requests
try:
response = requests.get('https://example.com')
response.raise_for_status()
except requests.exceptions.RequestException as e:
print(f"An error occurred: {e}")
Basic Syntax of Try-Except
Understanding the basic syntax of the try-except block in Python is essential for anyone looking to handle exceptions and errors gracefully. Let’s break down how this block works and look at a simple example to illustrate its usage.
Explanation of Try and Except Keywords
The try-except block is Python’s built-in mechanism for catching and handling exceptions. It helps prevent your program from crashing when an error occurs and allows you to manage the error in a controlled way. The structure of a try-except block is straightforward:
- try: This keyword is used to define a block of code that you want to test for errors.
- except: This keyword is used to define a block of code that will execute if an error occurs in the try block.
Here’s a simple diagram of the syntax:
try:
# Code that might raise an exception
except ExceptionType:
# Code that runs if the exception occurs
Code Example
Suppose you have a simple program that divides two numbers, but you want to ensure it doesn’t crash if someone tries to divide by zero:
try:
numerator = 10
denominator = 0
result = numerator / denominator
except ZeroDivisionError:
print("Error: Cannot divide by zero!")
In this example:
- The
try
block contains the code that attempts to dividenumerator
bydenominator
. - The
except ZeroDivisionError
block catches the specificZeroDivisionError
that occurs when you try to divide by zero and prints an error message instead of allowing the program to crash.
How It Works
- Execution of Try Block: Python first executes the code within the
try
block. If there are no errors, it skips theexcept
block entirely. - Catching Exceptions: If an error occurs during the execution of the
try
block, Python immediately jumps to theexcept
block. It checks if the type of error matches the exception specified. If it does, the code inside theexcept
block runs. - Skipping Remaining Try Block: If an error is caught, the rest of the
try
block is skipped, and Python proceeds with the next part of the program after theexcept
block.
Key Points to Remember
- Specificity: You can catch specific types of exceptions by specifying them after the
except
keyword (e.g.,except ZeroDivisionError
). - Multiple Except Blocks: You can have multiple
except
blocks to handle different types of exceptions separately. - Generic Exception: If you want to catch any exception, you can use
except Exception
without specifying an exception type, but this is generally not recommended as it can make debugging more difficult.
Using Else and Finally Clauses
In Python, the try-except
block is a powerful tool for handling exceptions and ensuring your program runs smoothly even when unexpected errors occur. Beyond the basic try
and except
keywords, Python offers additional clauses like else
and finally
that can enhance your error-handling strategy. Understanding and effectively using these clauses can make your code more robust and easier to maintain.
Purpose of the Else Clause
The else
clause in a try-except
block is optional but highly useful. It allows you to specify a block of code that will run only if no exceptions were raised in the try
block. Essentially, it helps you separate the code that should run when everything goes as expected from the code that should handle exceptions.
Syntax:
try:
# Code that might raise an exception
except SomeException:
# Code that runs if an exception occurs
else:
# Code that runs if no exception occurs
Example:
try:
result = 10 / 2
except ZeroDivisionError:
print("Cannot divide by zero.")
else:
print("Division successful. Result:", result)
In this example, the else
clause runs only if the division operation does not raise a ZeroDivisionError
. If the division is successful, the message “Division successful. Result: 5.0” is printed. If an exception occurs, the else
block is skipped.
Purpose of the Finally Clause
The finally
clause is another optional part of a try-except
block, but it serves a different purpose. The code within a finally
block will always run, regardless of whether an exception was raised or not. This is particularly useful for cleanup actions that you want to ensure are executed, such as closing files or releasing resources.
Syntax:
try:
# Code that might raise an exception
except SomeException:
# Code that runs if an exception occurs
else:
# Code that runs if no exception occurs
finally:
# Code that always runs, no matter what
Example:
try:
file = open('example.txt', 'r')
content = file.read()
except FileNotFoundError:
print("File not found.")
else:
print("File read successfully.")
finally:
file.close()
print("File closed.")
This is crucial for resource management and preventing resource leaks.
Combining Else and Finally
You can use both else
and finally
clauses together to create a comprehensive error-handling structure that separates the logic of successful execution, error handling, and cleanup.
Example:
try:
file = open('example.txt', 'r')
content = file.read()
except FileNotFoundError:
print("File not found.")
else:
print("File read successfully.")
finally:
file.close()
print("File closed.")
In this combined example, the else
block runs only if no exceptions are raised, and the finally
block runs no matter what, ensuring the file is closed in all cases.
Real-World Examples of Try-Except Blocks
Let’s dive into some real-world examples to see how try-except blocks are used in practical scenarios. Understanding these examples will give you a better grasp of how to implement error handling in your own projects.
Example 1: File Handling
Imagine you are writing a script to read data from a file. There are many potential points of failure here: the file might not exist, you might not have the necessary permissions, or the file could be corrupted. Using a try-except block can help handle these errors gracefully.
try:
with open('data.txt', 'r') as file:
data = file.read()
print(data)
except FileNotFoundError:
print("The file does not exist.")
except PermissionError:
print("You do not have permission to read this file.")
except Exception as e:
print(f"An error occurred: {e}")
Explanation:
- try: The block of code that attempts to open and read the file.
- except FileNotFoundError: If the file is not found, this block executes, printing an appropriate message.
- except PermissionError: If there is a permissions issue, this block handles it.
- except Exception as e: A catch-all for any other exceptions, ensuring the program does not crash and provides an error message.
Example 2: Network Requests
In applications that interact with web services, network requests can fail for various reasons, such as network connectivity issues, server downtime, or invalid URLs. Using try-except blocks allows you to manage these scenarios and provide meaningful feedback or retry logic.
import requests
url = "https://api.example.com/data"
try:
response = requests.get(url)
response.raise_for_status() # Raises an HTTPError for bad responses
data = response.json()
print(data)
except requests.exceptions.HTTPError as http_err:
print(f"HTTP error occurred: {http_err}")
except requests.exceptions.ConnectionError:
print("Error connecting to the server. Please check your internet connection.")
except requests.exceptions.Timeout:
print("The request timed out. Try again later.")
except requests.exceptions.RequestException as req_err:
print(f"An error occurred: {req_err}")
Explanation:
- try: The block where the network request is made.
- response.raise_for_status(): This method raises an HTTPError if the HTTP request returned an unsuccessful status code.
- except requests.exceptions.HTTPError as http_err: Handles HTTP errors and provides a specific message.
- except requests.exceptions.ConnectionError: Catches connection errors, typically due to network issues.
- except requests.exceptions.Timeout: Manages timeout errors, suggesting the user try again later.
- except requests.exceptions.RequestException as req_err: A generic catch-all for any request-related errors, ensuring no unhandled exceptions.
These examples illustrate how try-except blocks can make your code more resilient and user-friendly by providing clear error messages and handling unexpected situations gracefully.
Common Mistakes to Avoid
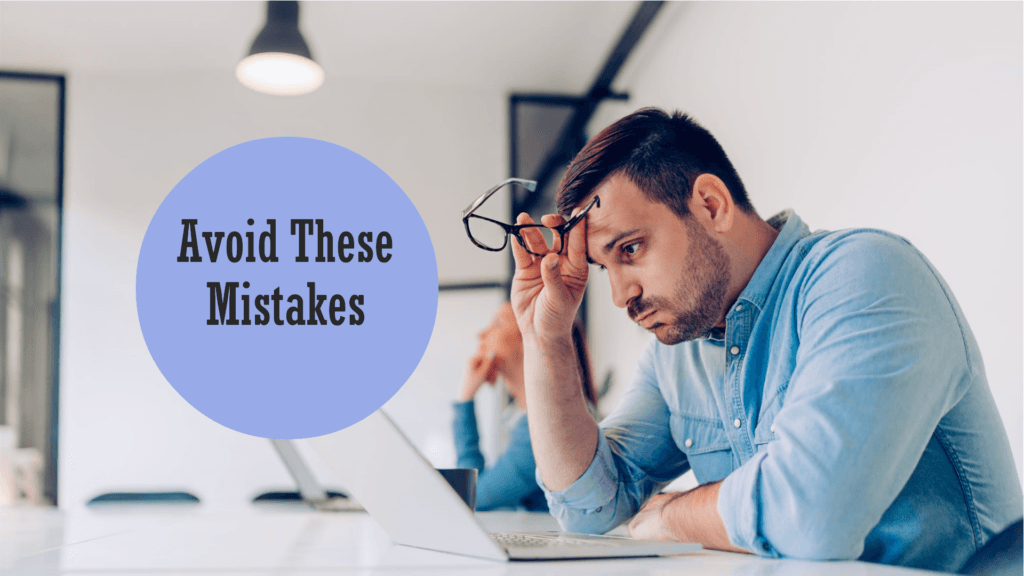
1. Overusing Try-Except Blocks
One of the most common mistakes is using try-except blocks excessively. While they are powerful tools for handling errors, they should not be used to control the flow of your program in normal operation. Using try-except blocks too often can make your code harder to read and maintain.
Example of Overuse:
try:
value = int(input("Enter a number: "))
except ValueError:
print("Invalid input. Please enter a number.")
In this case, it might be better to validate the input before converting it to an integer, rather than relying on try-except to handle every possible error.
2. Catching Broad Exceptions
Another common mistake is catching broad exceptions like Exception
or BaseException
. This approach can mask other types of errors that you didn’t intend to catch, making debugging more difficult. It’s better to catch specific exceptions so that you can handle each one appropriately.
Bad Example:
try:
risky_function()
except Exception:
print("An error occurred.")
Good Example:
try:
risky_function()
except ValueError:
print("A ValueError occurred.")
except TypeError:
print("A TypeError occurred.")
3. Ignoring Exceptions
Sometimes, developers catch exceptions without doing anything about them, which is effectively the same as ignoring the error. This practice can lead to silent failures, where your program doesn’t work as expected, but you have no idea why.
Example of Ignoring Exceptions:
try:
risky_function()
except ValueError:
pass # This ignores the ValueError
It’s important to handle the exception or at least log it so you can address the issue.
4. Using Try-Except for Flow Control
Using try-except blocks to control the normal flow of your program is not a good practice. Try-except should be used for handling exceptional situations, not for regular control flow.
Example of Bad Flow Control:
def is_integer(value):
try:
int(value)
return True
except ValueError:
return False
Instead, you should check conditions explicitly:
Better Approach:
def is_integer(value):
return value.isdigit()
5. Not Logging Exceptions
When exceptions are caught and handled, it’s important to log them. Logging exceptions helps you understand what went wrong and can be invaluable for debugging and maintaining your code.
Example Without Logging:
try:
risky_function()
except ValueError:
print("An error occurred.")
Example With Logging:
import logging
try:
risky_function()
except ValueError as e:
logging.error(f"A ValueError occurred: {e}")
6. Catching and Reraising Exceptions Incorrectly
If you catch an exception and intend to re-raise it, make sure you use the raise
statement correctly. Using raise
without an argument re-raises the current exception, which is useful for adding context while preserving the stack trace.
Incorrect Reraise:
try:
risky_function()
except ValueError as e:
raise e # This loses the original traceback
Correct Reraise:
try:
risky_function()
except ValueError:
raise # This preserves the original traceback
7. Not Using Finally for Cleanup
When you have resources that need to be cleaned up (like files or network connections), ensure you use the finally
block to guarantee the cleanup happens, regardless of whether an exception was raised.
Example Without Finally:
file = open("example.txt", "r")
try:
data = file.read()
except IOError:
print("An IOError occurred.")
file.close() # This might not be executed if an exception occurs
Example With Finally:
file = open("example.txt", "r")
try:
data = file.read()
except IOError:
print("An IOError occurred.")
finally:
file.close() # This will always be executed
Conclusion
Throughout this article, we’ve explored the fundamentals of the try-except block in Python, from its basic syntax to more advanced topics like handling multiple exceptions and using custom exception classes.
Error handling is an essential skill for any programmer. By using try-except blocks effectively, you can ensure your programs run smoothly even when unexpected errors occur, improving reliability and user satisfaction.
As with any programming concept, the best way to master try-except blocks is through practice. Try incorporating them into your projects to see how they can help you manage errors gracefully.
Don’t stop here! Continue to explore Python’s rich set of error-handling features and apply what you’ve learned to your coding projects. The more you practice, the more adept you’ll become at writing robust, error-resistant programs.
FAQs – Frequently Asked Questions
How do you handle exceptions in Python?
Handling exceptions in Python involves using the try-except block. You place the code that might raise an exception inside the try block, and then you write code in the except block to handle the exception if it occurs. Here’s a simple example:
try:
result = 10 / 0
except ZeroDivisionError:
print("You can't divide by zero!")
In this example, the code inside the try block will raise a ZeroDivisionError
because dividing by zero is not allowed. The except block catches this exception and prints a friendly error message instead of crashing the program.
What is the difference between try-except and try-finally?
The try-except block is used to catch and handle exceptions, allowing the program to continue running even if an error occurs. The try-finally block, on the other hand, is used to execute code regardless of whether an exception is raised or not. The finally block is always executed, making it useful for cleanup actions like closing files or releasing resources.
Example of try-finally:
try:
file = open('example.txt', 'r')
content = file.read()
finally:
file.close()
In this example, the file is closed whether an exception occurs or not.
Can you have multiple except blocks for a single try block?
Yes, you can have multiple except blocks to handle different types of exceptions separately. This allows you to provide specific responses to different error conditions.
Example:
try:
value = int(input("Enter a number: "))
result = 10 / value
except ValueError:
print("That's not a valid number!")
except ZeroDivisionError:
print("You can't divide by zero!")
In this example, if the user enters a non-integer value, a ValueError
will be caught. If the user enters zero, a ZeroDivisionError
will be caught.
What is the else clause in try-except blocks?
It is useful for code that should only run if the try block was successful.
Example:
try:
value = int(input("Enter a number: "))
result = 10 / value
except ZeroDivisionError:
print("You can't divide by zero!")
else:
print("The result is:", result)
Here, if no exception is raised, the else block prints the result.
How does the finally clause work in Python’s try-except blocks?
The finally clause is always executed after the try and except blocks, regardless of whether an exception was raised or not.
Example:
try:
file = open('example.txt', 'r')
content = file.read()
except FileNotFoundError:
print("The file was not found.")
finally:
file.close()
In this example, the file is closed no matter what, ensuring that resources are properly released.