Welcome to Technology Moment, where we explore the latest trends, insights, and best practices in the ever-evolving world of technology. In today’s fast-paced digital landscape, staying ahead requires not only understanding emerging technologies but also mastering the fundamental practices that drive success in software development.
In our blog on the “Top 10 Software Development Best Practices,” we’ll dive deep into the essential principles that every developer and tech team should adopt. From writing clean code to implementing effective unit testing and conducting thorough code reviews, these practices are vital for creating high-quality, maintainable software. Whether you’re a seasoned professional or just starting your journey in software development, our insights will help you enhance your skills and improve your projects.
Join us as we navigate the best practices that not only elevate individual performance but also foster collaboration and innovation within teams. Let’s unlock the potential of software development together and pave the way for a more efficient, productive, and successful tech environment.
Software development best practices are guidelines or methods that ensure the creation of high-quality software. These practices aren’t just about coding; they cover the entire development process, from the initial idea to the final deployment and beyond. Following these practices helps developers work more efficiently, reduce bugs, and create software that is easier to maintain and scale over time.
Why are best practices so important? The software development process is complex and involves multiple phases, including planning, designing, coding, testing, and maintaining the software. If a developer doesn’t follow best practices, the code can become messy, difficult to understand, and full of bugs. This not only slows down development but also makes it harder for other developers to collaborate or take over the project. On the flip side, following well-established best practices ensures a smoother development cycle, with fewer issues and faster delivery times.
These best practices act as a roadmap for developers, giving them a clear set of rules to follow. For instance, they guide how to write code that is clean, easy to read, and adaptable for future updates. They also cover collaborative activities like code reviews and documentation, which ensure that the entire team can contribute and understand the codebase.
Software development is not static; as technology evolves, so do the best practices. Developers need to stay updated with the latest tools and techniques to remain efficient and competitive. A focus on security, automation (like Continuous Integration/Continuous Deployment), and testing has become essential in modern development environments. Incorporating these best practices leads to more reliable and scalable software that meets business and user needs.
In short, adopting software development best practices ensures that you produce software that not only functions well but can also evolve and improve over time.
Why Following Best Practices Is Crucial for Success
Following best practices in software development is essential for ensuring the long-term success of projects. These best practices act as a roadmap, guiding developers toward efficient, maintainable, and scalable solutions. Here’s why adhering to them is so important:
1. Improves Code Quality: Best practices are designed to improve the quality of code. Writing clean, well-structured code reduces bugs, makes debugging easier, and ensures that the code is readable by other developers. This is crucial for projects that are worked on by multiple developers or teams, as it allows for better collaboration and understanding.
2. Enhances Maintainability: Following best practices ensures that your code is easily maintainable. Well-organized and documented code is much easier to update, refactor, or modify. This helps reduce technical debt and keeps the software running smoothly over time.
3. Reduces Bugs and Errors: When developers follow best practices such as unit testing, continuous integration (CI), and code reviews, the likelihood of introducing bugs into the software is greatly reduced. Automated testing catches issues early in the development process, and code reviews provide a second layer of oversight, preventing common mistakes that could lead to larger problems down the line.
4. Facilitates Team Collaboration: In large software projects, multiple developers or teams often work together on different aspects of the codebase. Following established best practices, such as consistent coding standards and proper documentation, ensures that everyone is on the same page. It makes onboarding new team members easier and ensures that existing members can collaborate efficiently without confusion or miscommunication.
5. Speeds Up Development: While it may seem counterintuitive, adhering to best practices can actually speed up the development process. By preventing major issues and making code easier to work with, developers spend less time fixing problems and more time building new features. Practices like CI/CD (Continuous Integration/Continuous Deployment) automate much of the testing and deployment process, ensuring that changes can be implemented quickly and efficiently.
6. Increases Scalability: As projects grow in size and complexity, following best practices ensures that the software remains scalable. Clean, modular code can be more easily adapted and extended to meet new requirements. Scalable software can handle increased loads, new features, and more users without needing complete rewrites or major overhauls.
7. Builds a Strong Foundation for Future Projects: Adhering to best practices sets a strong foundation for future development. Whether it’s a small bug fix or a major feature addition, developers working on the project in the future will appreciate that the code is clean, well-documented, and follows a clear set of guidelines. This creates a smoother development experience and ensures long-term success.
8. Improves Security: Security is a critical aspect of software development, and following security best practices helps mitigate risks such as data breaches, unauthorized access, and malicious attacks. By incorporating security measures into the development process—such as secure coding guidelines, regular security audits, and the principle of least privilege—developers can minimize vulnerabilities and create safer software.
9. Ensures Compliance with Industry Standards: In certain industries, such as healthcare or finance, software must adhere to strict regulations and standards. Following best practices ensures compliance with these industry-specific requirements. This not only helps avoid legal issues but also builds trust with customers and stakeholders, as they can be confident that the software meets high-quality standards.
10. Provides a Competitive Advantage: Finally, following best practices gives organizations a competitive edge. By consistently delivering high-quality, secure, and efficient software, companies can build a reputation for reliability and excellence. This can attract more customers, partners, and investors, ultimately driving business success.
Table of Contents
Best Practice #1: Writing Clean Code
Writing clean code is one of the fundamental best practices in software development. Clean code not only makes the development process smoother but also ensures that your codebase is easier to maintain, read, and scale over time.
What is Clean Code?
Clean code is code that is simple, clear, and understandable. It should be written in a way that another developer (or even your future self) can easily comprehend, modify, or fix without a steep learning curve. Clean code avoids unnecessary complexity and focuses on readability, maintainability, and simplicity.
Think of clean code as the “good hygiene” of software development—just like you maintain your health by following hygiene practices, you maintain the health of your codebase by writing clean, well-structured code.
Benefits of Clean Code
Writing clean code brings numerous advantages to both developers and organizations:
- Readability: Code is read more often than it is written, and clean code is easy to read and understand. When your code is readable, other developers can quickly grasp what it does without confusion.
- Maintainability: Over time, software evolves. Clean code ensures that these changes can be made easily, whether you’re adding features or fixing bugs. A clean codebase is less likely to introduce errors when updates are made.
- Collaboration: In a team environment, clean code is crucial. When multiple developers work on the same project, they need to quickly understand each other’s work. Clean code reduces the friction of collaboration and code review processes.
- Efficiency in Debugging: Clean code often has fewer bugs. And when bugs do appear, they are easier to track down and fix because the code is logically structured.
- Scalability: Clean code is typically modular and follows good design principles, making it easier to extend and scale as your project grows.
Tips for Writing Clean Code
Here are some essential tips to help you write cleaner code:
- Use Meaningful Names for Variables, Functions, and Classes: One of the core aspects of clean code is naming conventions. Use descriptive and meaningful names that clearly describe the purpose of the variable or function. Avoid abbreviations or vague names like
x
ortemp
, which make it harder to understand what your code is doing.- Example:
# Instead of:
x = calculate(a, b)
# Use:
total_cost = calculate_total_cost(product_price, quantity)
- Keep Functions and Methods Small: Functions and methods should focus on doing one thing and doing it well. Long, multi-tasking functions are harder to read and test. By breaking them down into smaller, single-purpose functions, you improve readability and reusability.
- Example:
# Rather than having an all-purpose function:
def process_order():
get_customer_info()
validate_payment()
calculate_shipping_cost()
send_confirmation_email()
# Break it down:
def process_order():
get_customer_info()
handle_payment()
finalize_order()
- Avoid Deep Nesting: Deeply nested code, such as loops within loops or multiple levels of if-else blocks, can quickly become hard to read and maintain. Instead, try to flatten your code by breaking it into smaller, separate functions.
- Write Comments Sparingly and Wisely: While comments are useful for explaining why certain decisions were made, they should not be used to explain what the code is doing—that’s the job of clean code. If your code needs excessive comments to explain what it does, it’s probably not clean. Focus on making the code itself self-explanatory.
- Follow Consistent Formatting: Consistency in formatting is key to clean code. Use proper indentation, spacing, and line breaks to ensure that your code is easy to follow. Tools like linters or code formatters can help maintain consistency across the team.
- Example:
def process_order(customer, order):
if order.is_valid:
customer.update_order_history(order)
process_payment(order)
send_confirmation(order)
- Avoid Magic Numbers and Strings: “Magic numbers” are unnamed numerical constants embedded in your code. Replace these with named constants that explain their purpose.
- Example:
# Instead of:
if score > 80:
award_bonus()
# Use:
BONUS_THRESHOLD = 80
if score > BONUS_THRESHOLD:
award_bonus()
- Handle Errors Gracefully: Clean code anticipates potential issues and handles them properly. This means using proper error handling and avoiding abrupt program crashes. Use
try-except
blocks (or equivalents in other languages) to manage errors effectively.- Example :
try:
process_payment(order)
except PaymentError as e:
log_error(e)
notify_user("There was an issue processing your payment.")
- Adopt a Consistent Naming Convention: Whether you’re using camelCase, snake_case, or another naming convention, consistency is key. Sticking to one style across your entire codebase makes it easier to read and understand.
- Write Tests as You Write Code: Writing tests alongside your code helps ensure that your code works as intended. Unit tests in particular are essential for verifying that each part of your code functions correctly. Clean code and well-written tests go hand in hand.
- Refactor Often: Don’t be afraid to go back and clean up code that you’ve already written. Code refactoring (discussed in another best practice) is about improving the structure of existing code without changing its functionality. Continuous refactoring helps keep your code clean and efficient over time.
Best Practice #2: Code Reviews
Code reviews are a critical part of the software development process. They involve evaluating someone else’s code to ensure it meets certain standards and is free of errors. This practice not only helps improve the overall quality of the codebase but also fosters a collaborative learning environment among developers. Let’s explore the key reasons why code reviews are important and how to conduct them effectively.
Why Code Reviews Are Important
- Improving Code Quality:
Code reviews provide a fresh pair of eyes on the code, which helps catch issues such as bugs, security vulnerabilities, or inefficient implementations that the original developer might have overlooked. The feedback ensures that the code aligns with project standards and follows best practices. - Knowledge Sharing:
Code reviews create an opportunity for team members to learn from one another. When experienced developers review junior developers’ work, it helps the junior team members improve their coding skills. Similarly, it can work the other way around, where new members bring in fresh ideas or approaches that benefit the entire team. - Enforcing Consistency:
By reviewing each other’s code, developers ensure that the code follows the agreed-upon standards and guidelines. This consistency is essential for maintainability, especially when multiple people are working on the same codebase over a long period. - Catching Bugs Early:
Bugs found during a code review are cheaper and easier to fix than bugs found after the code has been deployed. Early detection of errors helps prevent costly downtime or the need for hotfixes later in the software development cycle. - Enhancing Collaboration:
Code reviews promote a culture of collaboration within the team. Developers discuss solutions, learn new techniques, and build trust. This collaborative environment leads to stronger, more cohesive teams where everyone feels responsible for the quality of the product.
How to Conduct Effective Code Reviews
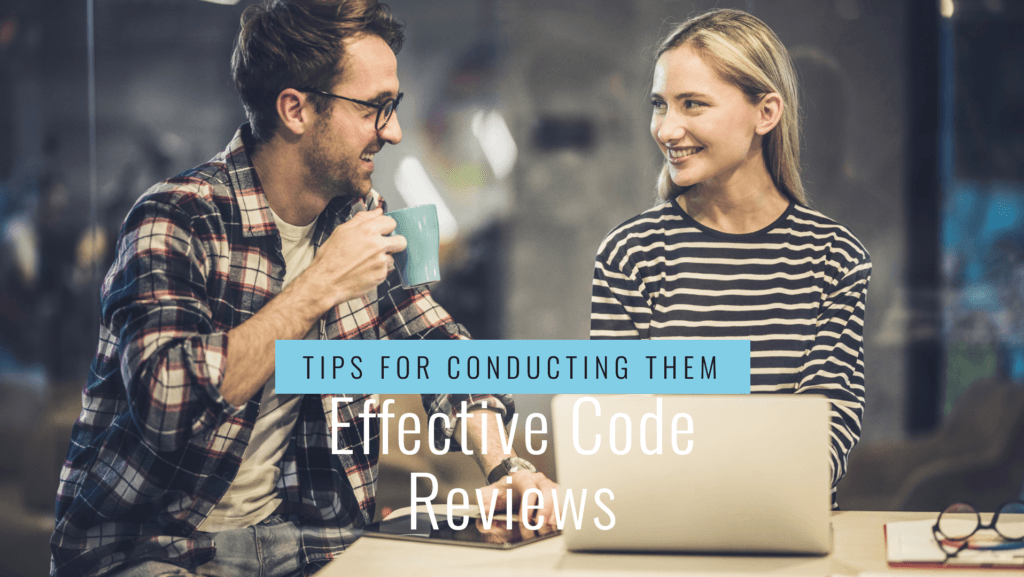
- Set Clear Guidelines:
Before starting code reviews, it’s essential to establish clear guidelines on what to look for. Are reviewers focused on functionality, performance, readability, security, or all of the above? Having these guidelines helps make reviews consistent and productive. - Review Small, Incremental Changes:
Reviewing large chunks of code at once can be overwhelming and lead to overlooked errors. It’s more effective to conduct reviews on smaller, incremental changes. This ensures the review process is thorough and less time-consuming, reducing the cognitive load on reviewers. - Focus on Key Aspects:
Reviewers should focus on the most critical aspects of the code, such as logic, structure, potential bugs, security vulnerabilities, and adherence to best practices. While reviewing, it’s also important to check if the code is easy to understand and maintain. - Provide Constructive Feedback:
The goal of a code review is to improve the quality of the code, not to criticize the person who wrote it. Feedback should be constructive, respectful, and aimed at helping the developer improve. It may be more efficient. - Use Automated Tools for Routine Checks:
Automated code review tools, such as linters and static analysis tools, can handle repetitive tasks like checking code formatting, style, or simple logic errors. This allows human reviewers to focus on more complex issues, such as the logic and architecture of the code. - Timebox the Review Process:
Code reviews shouldn’t take too long. Timeboxing the review process (for example, spending no more than 60 minutes on a review) ensures that the team remains productive and doesn’t spend excessive time on reviews. Quick, focused reviews help maintain momentum and reduce bottlenecks in development. - Encourage Open Communication:
Developers should feel comfortable asking questions or clarifying feedback during a review. Open communication ensures that both the reviewer and the author of the code understand each other’s perspective and can collaborate effectively to improve the code. - Celebrate Good Code:
Code reviews shouldn’t just be about finding faults. Positive feedback is equally important. Recognize and celebrate well-written, efficient, and innovative code. This helps boost morale and reinforces good coding practices.
Best Practice #3: Comprehensive Documentation
In the world of software development, documentation is one of the most important yet often overlooked aspects. While developers may focus on writing functional code, without proper documentation, maintaining and scaling the software becomes difficult. Comprehensive documentation serves as a roadmap for both the current development team and anyone who might work on the project in the future.
Why Documentation Matters
Documentation plays a crucial role in ensuring that everyone involved in a project understands how the software works, how it was built, and how it can be maintained or improved. It provides clarity for developers, testers, managers, and even clients. Whether it’s a new developer onboarding or a team member trying to fix a bug, well-written documentation can save time, reduce confusion, and minimize mistakes.
Here’s why it matters:
- Facilitates Understanding: Documentation ensures that team members can understand how different components of the software interact. It provides context, helping them make informed decisions when modifying the code.
- Speeds Up Onboarding: When new developers join a project, clear documentation allows them to get up to speed quickly. They won’t have to rely solely on senior developers to explain how everything works.
- Enhances Maintainability: Software isn’t static. Bugs need to be fixed, features need to be added, and sometimes, the code needs to be refactored. Documentation provides the necessary information to maintain and improve the software without requiring the original developers to be involved.
- Boosts Collaboration: For teams working across different locations or time zones, documentation serves as a single source of truth. It helps avoid misunderstandings and ensures everyone is on the same page.
Different Types of Documentation
Effective documentation isn’t a one-size-fits-all solution. It comes in various forms, each serving a different purpose:
- Code Documentation: This refers to comments within the code that explain what the code is doing and why certain decisions were made. It’s not just about what a function does but the reasoning behind how it’s written.
- API Documentation: If the software includes APIs, thorough documentation is essential. This typically includes how to authenticate, the structure of requests and responses, error handling, and usage examples.
- Technical Documentation: This is geared toward developers and includes architectural diagrams, design decisions, workflows, and the overall system structure.
- User Documentation: Aimed at end-users or clients, this type of documentation explains how to use the software. It may include user guides, manuals, or even instructional videos.
- Internal Documentation: This is documentation specific to the development process, including build instructions, environment setup, and any special tools or configurations required to work on the project.
Best Practices for Writing Documentation
Creating good documentation requires more than just writing out technical details.
- Write for Your Audience: Always keep in mind who will be reading the documentation. Documentation for developers should be technical and detailed, while user guides should be simpler and focused on usability.
- Keep It Clear and Concise: Avoid overly complex language or jargon that may confuse the reader. The goal is to communicate information in the simplest and most straightforward way possible.
- Update Regularly: Outdated documentation is as bad as no documentation at all. Make it a practice to update the documentation every time there is a change in the code, process, or system.
- Use Visual Aids: Diagrams, charts, and screenshots can help clarify complex topics. Visual aids make it easier to understand how different parts of the software interact and how to use it.
- Organize for Easy Navigation: Break the documentation into sections and use clear headings so readers can easily find the information they need. A table of contents or index can be especially helpful for larger projects.
- Automate Where Possible: For large projects, automated tools can be used to generate parts of the documentation, such as API references. This ensures consistency and accuracy.
- Keep It Versioned: If the software goes through multiple versions, make sure the documentation is clearly marked with version numbers. This prevents confusion when someone is looking at outdated information.
Best Practice #4: Code Refactoring
What is Code Refactoring?
It’s like renovating a house—you keep the structure intact, but you improve the design and quality of what’s inside. The goal is to enhance the code’s readability, efficiency, and maintainability while ensuring that it still functions as originally intended.
Refactoring is essential because, over time, software can become harder to understand or work with, especially if multiple developers are contributing to the same project. Poorly structured code can slow down development, increase bugs, and make it harder to introduce new features. Refactoring helps address these issues by keeping the codebase clean and organized.
Benefits of Refactoring Code
Refactoring brings several advantages to the software development process:
- Improved Code Readability: Cleaner, well-organized code is easier for developers (including your future self) to read and understand. This reduces the learning curve for new team members and makes debugging or adding features simpler.
- Easier Maintenance: Refactoring helps developers manage code changes more effectively. When code is well-organized, it’s easier to locate and fix bugs or update sections without causing unintended issues elsewhere.
- Enhanced Performance: Although refactoring doesn’t directly aim to improve performance, it often leads to optimized code paths. By simplifying complex structures or removing redundant logic, the program may run faster and use fewer resources.
- Reduction of Technical Debt: Technical debt refers to the implied cost of future rework caused by choosing quick and dirty solutions instead of a better, slower approach. Refactoring reduces technical debt, making it easier to expand and scale the software in the future.
- Increased Stability: Well-refactored code is less prone to errors and regressions. By eliminating duplication, consolidating similar functions, and adhering to design principles, you reduce the likelihood of introducing bugs when changes are made.
Best Times to Refactor Code
There are strategic moments when refactoring is especially beneficial:
- Before Adding New Features: If you’re planning to add new functionality to an existing codebase, refactoring can help make the code cleaner and easier to work with. This reduces the chances of new features introducing bugs or conflicts.
- After Fixing Bugs: Refactoring after bug fixes ensures that any messy or confusing code that led to the bug is cleaned up, preventing similar issues from arising in the future.
- During Code Reviews: Code reviews are an excellent opportunity to suggest refactoring improvements. Developers can identify areas where the code could be simplified or improved without impacting functionality.
- When Performance Drops: If your codebase is becoming slow or unresponsive, refactoring may help streamline inefficient code paths, improving performance.
- After Meeting Initial Project Milestones: Once a project reaches a stable point, it’s a good time to revisit the code and refactor, especially if you had to rush some parts to meet a deadline.
Common Refactoring Techniques
Here are some popular refactoring methods developers often use:
- Extract Method: Break a large function into smaller, reusable functions. This helps reduce repetition and makes the code easier to understand.
- Rename Variables and Functions: Give meaningful names to variables and functions so their purpose is immediately clear. Avoid cryptic or overly abbreviated names.
- Eliminate Redundant Code: Identify and remove any code that isn’t necessary or is repeated in multiple places. This helps reduce the overall size of the codebase and simplifies future updates.
- Simplify Conditional Logic: Refactor complex if-else or switch statements into simpler, more readable constructs. Use design patterns or polymorphism when appropriate.
- Replace Magic Numbers with Constants: Hardcoded values (magic numbers) can be confusing and error-prone. Refactor them into named constants for better clarity.
Challenges of Code Refactoring
- Risk of Introducing Bugs: Even though refactoring aims to preserve functionality, there’s always a risk of introducing bugs, especially if you don’t have sufficient unit tests in place to verify the changes.
- Time-Consuming: Refactoring can take time, which might delay other tasks, especially in projects with tight deadlines. It’s essential to balance refactoring efforts with other priorities.
- Lack of Immediate Payoff: The benefits of refactoring may not be visible immediately. This can make it hard to justify the time spent refactoring, especially to stakeholders who expect quick results.
- Difficulty in Recognizing When to Refactor: It’s not always easy to determine when code needs refactoring, especially if the project is progressing smoothly. Refactoring too often can also waste time, so it’s essential to refactor at the right moments.
How to Approach Refactoring
- Test First: Before starting any refactoring, ensure you have a comprehensive suite of tests. Unit tests, integration tests, and automated testing tools can help catch issues introduced during the refactoring process.
- Refactor in Small Steps: Don’t try to refactor everything at once. Focus on small, incremental changes to minimize the risk of errors. Tackle one section of code at a time, test it, and then move on to the next.
- Collaborate with the Team: If you’re working in a team, communicate your refactoring plans and ensure everyone is on board. Code reviews and peer feedback can help improve the quality of the refactor and catch potential issues early.
- Monitor Performance: While refactoring is generally focused on improving code quality, it’s still important to monitor the performance of your application during the process to ensure it doesn’t degrade.
Best Practice #5: Unit Testing
Unit testing is a fundamental software development practice that ensures the reliability and quality of code by testing individual units or components of a software application. These “units” are typically the smallest pieces of code that can be isolated for testing, such as functions, methods, or classes. By testing each unit separately, developers can identify bugs and errors early in the development process, making it easier to fix issues before they escalate.
Importance of Unit Testing
Unit testing plays a critical role in maintaining the quality of software, as it allows developers to verify that each part of the code works as expected. This is especially important in complex projects where different components interact with each other. If a single unit fails, it could affect other parts of the system, leading to major issues in the long run. Here are a few key reasons why unit testing is essential:
- Early Bug Detection: Unit testing helps catch bugs early, during the development phase. This reduces the likelihood of bugs making it into the final product, saving time and resources in debugging later.
- Code Reliability: Unit tests provide a safety net that ensures new changes don’t break existing functionality. Every time a developer modifies code, the unit tests can be rerun to check for any negative impacts.
- Simplifies Refactoring: When a solid suite of unit tests exists, developers can confidently refactor and optimize code knowing that the tests will detect if something goes wrong.
- Documentation of Code Behavior: Unit tests act as a form of documentation, describing how different parts of the code are supposed to behave. This can be helpful for new developers who join a project or for future reference.
What Makes a Good Unit Test?
A good unit test is one that is focused, isolated, and repeatable. It should test only one specific behavior or function of a unit and should not depend on external factors like databases or APIs. Here are some characteristics of effective unit tests:
- Isolated: A unit test should test only the specific function it’s intended to. This isolation ensures that the test results are not influenced by external factors.
- Repeatable: Tests should yield the same results every time they are run, regardless of the environment or other changes in the system.
- Readable: Unit tests should be easy to understand. Developers should be able to quickly glance at a test and know what it’s checking for. Clear and concise naming conventions for test cases help with this.
- Fast: Unit tests should be quick to run. Since they are typically executed frequently, tests that take too long can slow down the development process.
- Consistent Results: Unit tests should always return the same result for the same input. This ensures reliability and prevents false positives or negatives.
Best Practices for Writing Unit Tests
To make the most out of unit testing, developers should follow certain best practices to ensure the effectiveness and maintainability of their test suites. Here are some practical tips:
- Test One Thing at a Time: Each unit test should focus on one specific functionality of the unit. Trying to test too many things at once can lead to confusion and make it harder to pinpoint issues.
- Use Descriptive Names: Test cases should have clear, descriptive names that indicate what functionality is being tested. For example, a function that tests if a user is successfully authenticated might be named
testUserAuthenticationSuccess
. - Write Tests as You Write Code: Unit tests should be written alongside the code they test. This practice ensures that testing becomes an integral part of the development process and avoids the temptation to skip testing later.
- Mock External Dependencies: Since unit tests should be isolated, it’s important to mock or simulate external systems like databases, APIs, or file systems. This ensures that tests run independently of external changes or failures.
- Aim for High Coverage, But Don’t Overdo It: Code coverage tools help measure the percentage of your code that is covered by unit tests. While high test coverage is generally a good thing, it’s not necessary to aim for 100% coverage. Instead, focus on testing critical paths and high-risk areas of the code.
- Use Automation Tools: Modern development environments provide a variety of tools to automate unit testing. Continuous Integration (CI) systems can automatically run tests every time code is pushed, making it easier to catch issues early.
- Refactor Tests When Necessary: Just like production code, unit tests also need to be refactored over time. As your application evolves, certain tests may become outdated or irrelevant. Regularly review your tests to ensure they are still relevant and useful.
Benefits of Unit Testing
By incorporating unit testing into the development process, teams can experience a range of benefits:
- Improved Code Quality: Well-tested code is often cleaner, more reliable, and easier to maintain.
- Faster Debugging: Since unit tests pinpoint exactly which part of the code has failed, debugging becomes more efficient.
- Reduced Costs: Finding and fixing bugs during the unit testing phase is significantly less costly than dealing with them after the product has been released.
- Facilitates Agile Development: Unit tests help in iterative and incremental development, allowing teams to deliver small, bug-free features regularly.
Unit testing serves as a powerful tool to ensure code quality, reduce bugs, and improve overall efficiency in the software development lifecycle. Following the best practices mentioned above will help you write better, more maintainable unit tests that contribute to a robust and reliable application.
Best Practice #6: Continuous Deployment (CD) and Continuous Integration (CI)
Continuous Integration (CI) and Continuous Deployment (CD) are vital processes in modern software development. Together, they streamline the development lifecycle by automating key aspects of software delivery, ensuring that changes made to the codebase are smoothly integrated, thoroughly tested, and quickly deployed into production environments.
The Role of CI/CD in Modern Development
CI/CD is all about improving collaboration, reducing errors, and accelerating the speed at which developers can release software. In traditional development workflows, integrating new code changes, running tests, and deploying updates were often time-consuming and prone to mistakes. However, CI/CD automates much of this work, enabling teams to develop and deliver software faster and with fewer bugs.
- Continuous Integration (CI) involves the practice of frequently merging all developer working copies to a shared mainline. Each integration is automatically verified by running tests, ensuring that the code is functional and doesn’t break any existing features.
- Continuous Deployment (CD) takes the process a step further, ensuring that after code passes testing, it is automatically deployed to production or staging environments. This ensures a quicker and more reliable deployment process.
Key Benefits of CI/CD
- Faster Development Cycles: By integrating code more frequently and automatically testing it, CI/CD helps developers identify and fix problems quickly, leading to shorter development cycles.
- Higher Code Quality: Automated testing is a key part of CI/CD pipelines. This ensures that any code integrated into the project passes a suite of tests, reducing the likelihood of bugs making it into production.
- Reduced Manual Errors: Because much of the process is automated, there is less room for human error. Releasing new software versions becomes predictable and smooth.
- Better Collaboration: With frequent integration, teams can work more cohesively, reducing communication gaps.
- Improved End-User Experience: Faster, more reliable software releases mean users get access to features, improvements, and bug fixes more quickly, improving overall user satisfaction.
Best Practices for Implementing CI/CD
- Automated Testing at Every Stage: The backbone of any CI/CD pipeline is automated testing. You should ensure that tests are run at every stage of the pipeline, including unit tests, integration tests, and performance tests. Automated testing helps catch issues early and prevents broken code from being deployed.
- Frequent Small Releases: Instead of waiting to release large batches of changes, adopt a strategy of frequent, small releases. This reduces the risk associated with each release and allows teams to deploy improvements and bug fixes faster.
- Use Version Control Properly: Your CI/CD pipeline should be tightly integrated with your version control system (e.g., Git). Every change in the code should trigger the pipeline process—compiling, testing, and deploying the software.
- Maintain a Stable Main Branch: In CI/CD, the main branch of your repository (often called
main
ormaster
) should always be in a deployable state. Developers should only merge their code into the main branch when it passes all tests. - Monitor and Rollback Strategies: While CI/CD automates deployment, it’s essential to have proper monitoring in place to detect issues post-deployment. Additionally, an easy rollback strategy is crucial in case a deployment introduces bugs or issues into production. This allows you to quickly revert to the previous stable version.
- Containerization for Consistency: Using containerization tools like Docker can help ensure that your code runs consistently in different environments (development, testing, and production). Containers package your software and its dependencies together, reducing “it works on my machine” problems.
- Security Checks in the Pipeline: Security should not be an afterthought. Integrating security tests into your CI/CD pipeline helps catch potential vulnerabilities early, ensuring your software is not only functional but also secure.
Real-World Example of CI/CD Implementation
Let’s say you have a development team working on an e-commerce platform. Every time a developer makes a change (like adding a new feature or fixing a bug), that change is committed to a shared repository. With CI, the system automatically runs tests on this new code to ensure it integrates well with the existing codebase. Once the code passes these tests, the CD pipeline automatically deploys it to a staging environment, where further testing occurs. If everything looks good, the changes are deployed to production, making the new feature or fix available to users—sometimes in a matter of minutes.
Best Practice #7: Consistent Coding Standards
Coding standards are a set of guidelines and best practices that ensure code is written in a consistent, readable, and maintainable way. Following consistent coding standards in a software development project helps developers maintain clarity, collaborate more effectively, and minimize errors. Let’s break down why these standards are so important and how to establish them for your team.
Why Coding Standards Are Important
- Improved Readability: Consistent coding standards make the code easier to read, not only for the person who wrote it but also for anyone who may work on it in the future. Imagine coming back to your own code after six months or having a colleague pick it up—if the code is written in a consistent style, it’s much simpler to understand.
- Reduced Complexity: Coding standards help reduce unnecessary complexity. When everyone on the team writes code in a similar way, there is less cognitive load when trying to understand the logic. This consistency can make the process of identifying bugs or adding new features faster and more efficient.
- Streamlined Collaboration: In team environments, developers often work on different parts of the same project. If each developer writes code using their own style, it can create confusion and inconsistencies, leading to potential errors and longer review times. Consistent coding standards ensure everyone is on the same page, making collaboration smoother.
- Easier Maintenance: Well-maintained code is easier to fix and update. When you have clear coding guidelines, developers can spot issues more easily and make changes without fear of breaking existing functionality. Maintenance becomes less of a headache when the code follows a predictable structure.
- Enhanced Code Quality: When standards are enforced, there is less room for careless mistakes like misnamed variables, inconsistent indentation, or improper use of functions. This results in cleaner, higher-quality code that is more reliable and less prone to bugs.
How to Establish and Enforce Coding Standards
- Define the Standards Early: Establish coding standards at the beginning of the project. This should cover everything from naming conventions for variables and functions to how code is formatted (e.g., tabs vs. spaces). Make sure these guidelines are documented and accessible to everyone on the team.
- Use Linting Tools: Linting tools are programs that automatically check code for style and quality issues based on predefined rules. Tools like ESLint for JavaScript or Pylint for Python can enforce coding standards automatically, reducing the manual effort needed to review every line of code. These tools can be integrated into the CI/CD pipeline to ensure that code meets standards before it’s merged.
- Regular Code Reviews: Code reviews are a key part of maintaining consistent standards. During a review, team members can check whether the submitted code adheres to the project’s guidelines. This not only improves the quality of the code but also gives junior developers a chance to learn from more experienced team members.
- Provide Training and Guidelines: Sometimes, inconsistency arises from a lack of understanding of the coding standards. To prevent this, it’s a good idea to provide training, especially for new team members. You can also create detailed coding guidelines that explain the rationale behind each standard.
- Stick to the Agreed-Upon Standards: Once you’ve defined coding standards, stick to them! Encourage all team members to follow the guidelines and ensure there is accountability for deviating from them. However, allow some flexibility when there is a justifiable reason to deviate, as long as it’s documented and discussed within the team.
- Periodically Update the Standards: Coding practices evolve, and so should your standards. Periodically review your coding guidelines and update them to reflect new best practices, changes in technology, or feedback from the team. This ensures that your team is following the latest approaches and improving continuously.
Common Areas to Standardize
- Naming Conventions: Agree on how to name variables, functions, classes, and files. For instance, you might decide to use camelCase for variables (
myVariable
) and PascalCase for classes (MyClass
). This consistency ensures that names are predictable and convey their purpose clearly. - Formatting: Set rules for how code should be formatted, such as where to place curly braces, how to indent lines, and how long each line of code should be. Formatting standards ensure that the structure of the code is uniform throughout the project.
- Error Handling: Standardize how errors are handled across the codebase. This might include using consistent error messages, logging practices, or defining custom exceptions in certain situations. A clear approach to error handling ensures that issues are captured and addressed in a consistent way.
- Commenting: While code should generally be self-explanatory, there are cases where comments are necessary. Establish guidelines for when to use comments and what they should explain (e.g., complex logic, the reasoning behind certain decisions). Standardizing comments ensures they are helpful and not overused.
- Use of Libraries and Frameworks: Agree on which libraries or frameworks are acceptable and how they should be used. This avoids unnecessary duplication or misuse of third-party tools.
Examples of Popular Coding Standards
- PEP 8: A widely adopted Python coding standard that outlines guidelines for writing readable, maintainable Python code. It covers topics such as indentation, line length, and naming conventions.
- Google JavaScript Style Guide: A comprehensive set of coding standards for JavaScript that focuses on clarity, simplicity, and performance. It has specific guidelines for indentation, line wrapping, and code structure.
- Airbnb JavaScript Style Guide: Another popular JavaScript style guide that focuses on readability and consistency. It’s widely used in the industry and is a good starting point for teams looking to standardize their code.
Best Practice #8: Agile Development Practices
Agile development methods, which prioritize adaptability, teamwork, and client happiness, have completely changed the software development industry. Let’s delve deeper into what Agile is, its benefits, and how to implement Agile methodologies effectively within a team.
What is Agile Development?
Agile is a project management and software development approach that prioritizes delivering small, incremental improvements to software, allowing teams to respond quickly to changing requirements. Unlike traditional methodologies, which often rely on a rigid sequence of phases (like Waterfall), Agile promotes an iterative and incremental process. This means that instead of delivering a complete product at the end of a long development cycle, Agile teams work in short, time-boxed iterations (called sprints) that typically last 1 to 4 weeks.
- Individuals and interactions over processes and tools: Focusing on communication and collaboration within teams rather than strictly following predefined processes.
- Working software over comprehensive documentation: Prioritizing the delivery of functional software that meets user needs over extensive documentation.
- Customer collaboration over contract negotiation: Engaging customers and stakeholders throughout the development process to ensure their feedback shapes the product.
- Responding to change over following a plan: Emphasizing the ability to adapt to new requirements and changes rather than adhering to a fixed plan.
Benefits of Agile Methodologies
Adopting Agile practices can bring several advantages to a software development team:
- Enhanced Flexibility: Agile allows teams to adapt to changes in requirements even late in the development process, making it easier to respond to market trends and customer feedback.
- Increased Collaboration: Agile promotes regular communication and collaboration among team members, stakeholders, and customers, fostering a culture of teamwork and shared ownership.
- Faster Time-to-Market: By delivering incremental updates, Agile teams can get new features and improvements into users’ hands more quickly, allowing businesses to remain competitive.
- Higher Quality Software: Continuous testing and integration practices in Agile help identify and fix bugs early in the development cycle, resulting in a more reliable and higher-quality product.
- Improved Customer Satisfaction: Engaging customers throughout the development process ensures that the final product meets their needs and expectations, leading to higher satisfaction and loyalty.
How to Implement Agile in Your Team
Successfully implementing Agile practices requires commitment and adjustments to the team’s workflow. Here are some steps to effectively adopt Agile methodologies:
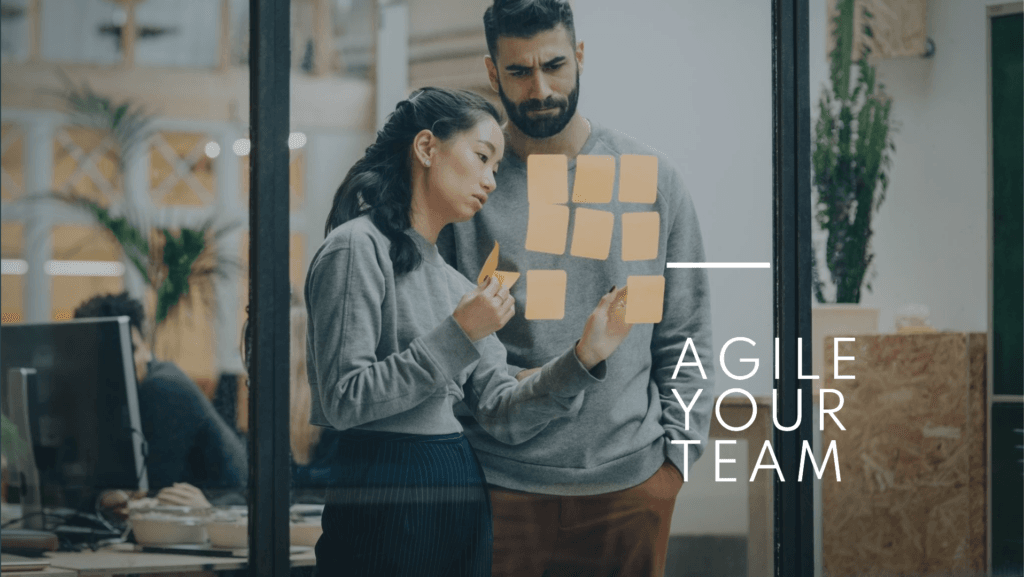
- Choose an Agile Framework: Select an Agile framework that suits your team’s needs. Popular frameworks include Scrum, Kanban, and Extreme Programming (XP). Each has its own practices and principles, so it’s essential to find the one that aligns best with your team’s goals and culture.
- Establish Cross-Functional Teams: Form teams composed of members with diverse skill sets (e.g., developers, designers, testers) to enhance collaboration and promote shared ownership of the project.
- Hold Regular Meetings: Schedule regular Agile ceremonies, such as daily stand-ups, sprint planning, sprint reviews, and retrospectives. These meetings facilitate communication, encourage feedback, and ensure everyone is aligned on project goals.
- Focus on User Stories: Use user stories to capture requirements from the user’s perspective. This approach helps the team prioritize features based on user needs and encourages a customer-centric mindset.
- Prioritize Backlog Management: Maintain a prioritized product backlog that outlines all tasks and features. Regularly refine and update the backlog to reflect changing priorities and new insights.
- Emphasize Continuous Improvement: After each sprint, conduct retrospectives to reflect on what went well and what could be improved. Use this feedback to adjust processes and enhance team performance.
- Encourage a Culture of Trust and Empowerment: Foster an environment where team members feel empowered to make decisions and voice their opinions. Trust is a vital component of successful Agile teams.
- Invest in Agile Training: Provide training for team members on Agile methodologies and best practices. Understanding the principles of Agile will help the team embrace the change more effectively.
Best Practice #9: Efficient Software Maintenance
Efficient software maintenance is a critical aspect of software development that ensures applications remain functional, secure, and relevant over time. It encompasses various tasks that range from fixing bugs and improving performance to implementing new features and ensuring compliance with the latest regulations. Let’s break down the importance of software maintenance, common challenges, and best practices for effective maintenance.
Why Software Maintenance Matters
- Extends Software Lifespan: Just like a car requires regular servicing to keep it running smoothly, software needs maintenance to continue functioning well. Efficient maintenance can significantly extend the lifespan of software applications.
- Adapts to Changing Requirements: As businesses evolve, so do their needs. Software must be regularly updated to reflect these changes, including adding new features or modifying existing ones.
- Ensures Security: With increasing cyber threats, maintaining software is crucial for identifying and patching security vulnerabilities. Regular updates help protect sensitive data and maintain user trust.
- Improves Performance: Over time, software can slow down or become less efficient due to accumulated data or outdated code. Regular maintenance helps optimize performance and improve user experience.
- Reduces Costs: Proactive maintenance is often more cost-effective than reactive measures. By identifying and fixing issues before they escalate, organizations can save on more extensive repairs or system failures.
Common Software Maintenance Challenges
- Legacy Code: Older software may rely on outdated technologies or coding standards, making it difficult to update or maintain. Understanding and working with legacy code can be a significant hurdle.
- Resource Limitations: Maintenance often requires dedicated time and personnel. Organizations may struggle to allocate resources effectively, leading to neglected maintenance tasks.
- Documentation Gaps: Poor documentation can hinder maintenance efforts. Without clear guidelines on the software’s architecture or previous updates, developers may find it challenging to implement changes.
- Resistance to Change: Teams may resist adopting new tools or practices that could improve maintenance efficiency. Overcoming this resistance is essential for implementing effective strategies.
- Balancing Maintenance and New Development: Organizations must strike a balance between maintaining existing software and developing new features. Prioritizing tasks can be challenging but is necessary for overall success.
Tips for Effective Software Maintenance
- Regular Updates: Schedule regular maintenance windows to update software, fix bugs, and improve performance. This practice helps prevent issues from becoming critical problems.
- Implement Version Control: Use version control systems like Git to track changes and manage updates effectively. This practice allows teams to collaborate and revert to previous versions if needed.
- Establish Coding Standards: Maintain consistent coding practices to make the codebase easier to understand and update. Clear standards can reduce technical debt and improve the overall quality of the code.
- Encourage Documentation: Ensure that all changes and maintenance tasks are well-documented. This practice helps future developers understand the rationale behind decisions and speeds up the maintenance process.
- Automate Where Possible: Automation can reduce human error, save time, and ensure consistent execution of maintenance tasks.
- Conduct Regular Code Reviews: Encourage team members to review each other’s code. This practice can help identify potential issues early, share knowledge, and improve code quality.
- Prioritize Technical Debt Management: Regularly assess and address technical debt in the codebase. Prioritizing the reduction of technical debt can lead to more efficient maintenance in the long run.
- User Feedback Integration: Collect and analyze user feedback to identify areas for improvement. Users often provide valuable insights into software performance and usability, which can guide maintenance efforts.
- Performance Monitoring: Use monitoring tools to track software performance in real time. Identifying performance bottlenecks early allows teams to address issues before they affect users.
- Create a Maintenance Plan: Develop a comprehensive maintenance plan that outlines tasks, timelines, and responsibilities. A structured approach helps teams stay organized and focused on maintenance activities.
Best Practice #10: Security Best Practices in Development
In today’s digital landscape, security is not just an add-on; it’s a fundamental aspect of software development. As software systems become increasingly complex and interconnected, they are also more vulnerable to attacks. This makes adhering to security best practices crucial for protecting sensitive data and maintaining user trust. Here, we’ll delve into the importance of security in software development, common vulnerabilities, and best practices to mitigate risks.
Why Security Should Be a Priority
Security should be a core component of every software development project for several reasons:
- Data Protection: Applications often handle sensitive data, including personal information and financial records. A security breach can lead to data theft, which can have devastating consequences for both users and companies.
- Compliance Requirements: Many industries are governed by regulations (like GDPR, HIPAA, etc.) that require organizations to implement strict security measures.
- Maintaining User Trust: A single security breach can lead to a loss of trust and customer loyalty, affecting a company’s reputation and bottom line.
- Cost of Breaches: The financial impact of a security breach can be significant. Costs can arise from remediation efforts, legal fees, regulatory fines, and lost business.
Common Security Vulnerabilities in Software
Before diving into best practices, it’s essential to understand the common vulnerabilities that developers face:
- Injection Attacks: SQL injection and other forms of injection attacks occur when an attacker sends untrusted data to a program, which can then execute malicious commands.
- Cross-Site Scripting (XSS): This vulnerability allows attackers to inject malicious scripts into webpages viewed by other users, potentially stealing sensitive information or hijacking user sessions.
- Cross-Site Request Forgery (CSRF): In a CSRF attack, a malicious website tricks a user’s browser into making requests to another site where the user is authenticated, potentially causing unwanted actions.
- Insecure Direct Object References (IDOR): This vulnerability occurs when an attacker can access unauthorized data or functions simply by modifying a URL or form field.
- Security Misconfigurations: Poorly configured security settings can leave applications and systems open to attacks. This can include default settings, unnecessary features, and overly permissive permissions.
- Sensitive Data Exposure: Failing to encrypt sensitive data or using weak encryption can lead to data being exposed during transit or at rest.
Best Practices for Secure Software Development
Implementing robust security practices in the software development lifecycle (SDLC) can significantly reduce risks. Here are some key practices to consider:
- Adopt a Security-First Mindset: Incorporate security considerations from the earliest stages of development. This includes threat modeling during the design phase and security requirements in the specifications.
- Conduct Regular Security Training: Developers should undergo regular security training to stay updated on the latest threats and best practices. This helps them recognize vulnerabilities and adopt secure coding habits.
- Use Secure Coding Guidelines: Follow established secure coding guidelines (like OWASP’s Top Ten) to address common security issues. Ensure that your team is familiar with these guidelines and applies them consistently.
- Implement Input Validation and Output Encoding: Validate all inputs to ensure they are correct and safe, and encode outputs to prevent injection attacks. For instance, always sanitize user inputs and escape outputs before rendering them in a web browser.
- Use Prepared Statements and Parameterized Queries: Protect against SQL injection by using prepared statements and parameterized queries.
- Implement Strong Authentication and Authorization: Use multi-factor authentication (MFA) to enhance security. Ensure that users have the least privilege necessary to perform their functions by implementing role-based access control (RBAC).
- Regularly Update and Patch Software: Keep all software, libraries, and dependencies up to date to protect against known vulnerabilities. Implement a schedule for regular updates and security patches.
- Conduct Code Reviews and Security Testing: Perform regular code reviews with a focus on security. Incorporate security testing (e.g., static and dynamic analysis) into your CI/CD pipeline to identify vulnerabilities before they reach production.
- Encrypt Sensitive Data: Ensure that sensitive information, like passwords and personal data, is stored securely.
- Develop an Incident Response Plan: Prepare for the worst by developing a robust incident response plan. This plan should outline how to respond to security breaches, including communication strategies and remediation efforts.
- Monitor and Audit Security: Continuously monitor your applications for security breaches and unusual activities.
Conclusion
In the fast-evolving landscape of technology, adhering to best practices in software development is not just beneficial; it’s essential for ensuring long-term success. As we’ve explored throughout this article, each best practice plays a crucial role in enhancing code quality, fostering collaboration among team members, and ultimately delivering a superior product to end users.
By prioritizing clean code, developers can significantly reduce technical debt, making it easier to maintain and extend the software in the future. Code reviews not only enhance code quality but also serve as a learning opportunity for developers, fostering a culture of shared knowledge and improvement.
Comprehensive documentation acts as a roadmap for both current and future developers, enabling them to navigate the codebase with ease and understand the rationale behind design decisions. This leads seamlessly into the practice of code refactoring, which ensures that code remains efficient and adaptable to changing requirements over time.
Implementing unit testing is paramount for catching bugs early, ensuring that each component functions as intended before integration. Coupled with a robust CI/CD pipeline, these practices streamline the development process, facilitating quicker releases while maintaining high standards of quality.
Establishing consistent coding standards across a team not only promotes readability but also helps to eliminate confusion and miscommunication among developers. Agile methodologies introduce flexibility and responsiveness into the development process, allowing teams to adapt to user feedback and changing market conditions effectively.
Moreover, the importance of efficient software maintenance cannot be overstated. Regular maintenance ensures that software continues to perform well and meets the evolving needs of users, minimizing disruptions and maximizing satisfaction. Lastly, prioritizing security best practices during development is crucial for protecting sensitive data and building trust with users.
In conclusion, adopting these top 10 software development best practices is a proactive step toward creating a resilient, high-quality software product. It sets a solid foundation for continuous improvement and innovation. By fostering a culture of excellence and collaboration, development teams can navigate the complexities of modern software development with confidence, ensuring that they not only meet but exceed user expectations.
Implementing these practices requires commitment and discipline, but the long-term benefits far outweigh the initial investment. As you reflect on your own development processes, consider which of these best practices you can integrate to enhance your team’s workflow and the overall quality of your software products.
FAQs – Frequently Asked Questions
What are software development best practices?
Software development best practices are a set of guidelines or principles that developers and teams follow to enhance the quality, efficiency, and maintainability of software projects. These practices encompass various aspects of software development, such as writing clean code, conducting code reviews, maintaining comprehensive documentation, performing unit testing, and ensuring security. By adhering to these best practices, teams can reduce bugs, improve collaboration, and deliver software that meets user needs more effectively.
Why is writing clean code important?
Writing clean code is essential because it enhances readability and maintainability. Clean code is easier for developers to understand, which reduces the time needed for onboarding new team members and makes it simpler to identify and fix bugs. It promotes consistent coding styles and practices, allowing for easier collaboration among team members. Additionally, clean code often leads to better performance and scalability, making it a crucial aspect of successful software development.
How can code reviews improve software quality?
Code reviews are an effective way to improve software quality by enabling team members to share knowledge and provide constructive feedback on each other’s work. During a code review, developers can identify potential issues, suggest improvements, and ensure that coding standards are met. This collaborative process helps catch bugs early, reduces technical debt, and encourages learning within the team. Moreover, it fosters a culture of accountability and continuous improvement, leading to higher-quality software in the long run.
What function does unit testing serve in the creation of software?
Unit testing plays a critical role in software development by allowing developers to test individual components or functions of the code in isolation. This practice helps identify and fix issues at an early stage, reducing the likelihood of bugs reaching production. Unit tests also serve as a form of documentation, demonstrating how the code is intended to work. By maintaining a robust suite of unit tests, developers can refactor code with confidence, knowing that any changes will be validated by these tests, ultimately leading to more stable and reliable software.
How can teams implement agile development practices?
Implementing agile development practices involves adopting a flexible and iterative approach to software development. Teams can start by embracing core agile principles such as collaboration, customer feedback, and responsiveness to change. This can be achieved through practices like daily stand-up meetings, sprint planning, and retrospective sessions to assess progress and identify areas for improvement. Tools like Scrum or Kanban can help manage workflows effectively. Additionally, teams should focus on delivering incremental value to users, ensuring that feedback is integrated into the development process to continuously enhance the software being built.